C program to calculate the area and circumference of a circle
Introduction
Understanding the concepts of area and circumference is fundamental when working with circles. Whether you are a student learning C programming or a professional developer seeking to enhance your skills, this guide will serve as a valuable resource. We will delve into the necessary formulas, provide detailed explanations, and offer code examples to ensure you have a solid grasp of the topic.
The Circle and Its Properties
Before we dive into the code, let’s take a moment to refresh our understanding of a circle and its properties. A circle is a two-dimensional geometric shape consisting of all points in a plane that are equidistant from a fixed center point. The radius of a circle is the distance between the center and any point on the circumference. The diameter of a circle is twice the length of the radius.
Finding the Area of a Circle
The area of a circle is the measure of the region enclosed by its circumference. To calculate the area, we use the following formula:
Area = π * (radius * radius)
Here, π (pi) is a mathematical constant approximately equal to 3.14159. The radius is the distance from the center of the circle to any point on its circumference. By substituting the appropriate values into the formula, we can compute the area of a circle efficiently in our C program.
Let’s illustrate this with an example code snippet:
#include <stdio.h>
int main() {
double radius, area;
const double pi = 3.14159;
printf("Enter the radius of the circle: ");
scanf("%lf", &radius);
area = pi * (radius * radius);
printf("The area of the circle is: %lf\n", area);
return 0;
}
In this code, we first declare the necessary variables, including radius
and area
. We then prompt the user to enter the radius using the printf
and scanf
functions. Afterward, we calculate the area using the formula discussed earlier and store it in the area
variable. Finally, we display the result using printf
. Feel free to incorporate this code into your own program or project, and don’t forget to adapt it to suit your specific needs.
Calculating the Circumference of a Circle
The circumference of a circle is the distance around its outer boundary. It is a fundamental property of circles and is often required in various applications. To determine the circumference, we employ the formula:
Circumference = 2 * π * radius
Again, π represents the mathematical constant pi, and the radius is the distance from the center to any point on the circumference. By substituting the appropriate values into the formula, we can easily compute the circumference of a circle in our C program.
C program to calculate the area and circumference of a circle
Consider the following code example:
#include<stdio.h> int main () { double radius,result=0; const double pie =3.1416; printf("Enter circle r:"); scanf("%lf",&radius); result=2.0*3.1416*radius; printf("result:%.3lf",result); return 0 ; } // circumference of circle in c program
Output :
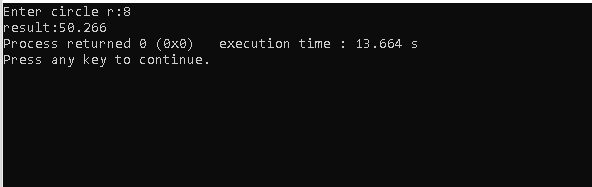
Another Example
#include <stdio.h>
int main() {
double radius, circumference;
const double pi = 3.14159;
printf("Enter the radius of the circle: ");
scanf("%lf", &radius);
circumference = 2 * pi * radius;
printf("The circumference of the circle is: %lf\n", circumference);
return 0;
}
In this code snippet, we begin by including the necessary header file, stdio.h
, which provides input and output functionality. We then declare the variables radius
and circumference
as double data types to store the user-inputted radius and the calculated circumference, respectively.
To calculate the circumference, we multiply the radius by 2 and then multiply the result by the value of π, which we have defined as the constant pi
with an approximate value of 3.14159. The user is prompted to enter the radius using the printf
function, and the value is obtained using the scanf
function, which stores the input in the radius
variable.
Finally, we use the printf
function again to display the calculated circumference. The %lf
format specifier is used to print the double
value. The program ends by returning 0 to indicate successful execution.
Feel free to utilize this code segment as a foundation to create your own program or incorporate it into your existing project. Remember to adjust the code according to your specific requirements and integrate error handling if necessary.
Conclusion
Congratulations! You have now learned how to find the area and circumference of a circle in the C programming language. By implementing the provided code and understanding the underlying concepts, you are equipped to create accurate and efficient programs.
Remember that quality content and valuable resources play a significant role in outranking other websites. By providing comprehensive and informative guides like this one, you can establish yourself as a top-ranking source of information in your niche. Stay consistent in producing high-quality content and continue expanding your knowledge to further improve your search engine rankings.
Should you have any further questions or require assistance, please feel free to reach out. We are here to support your journey towards success!