BEECROWD 1074 Even or Odd Solution in C,C++,Python
BEECROWD 1074 Even or Odd question
Welcome to our comprehensive guide on how to solve the “BEE CROWD 1074 Even or Odd ” problem using C++, C and Python. In this article, we will explain the solution to the Even Square problem, how to write code in C++, C and Python, and how to optimize the code for maximum performance.
Problem Description
The Even Square problem requires us to find the sum of the squares of even numbers between two given numbers, inclusive. For example, given the input range [2,10], the program should output 220, which is the sum of the squares of the even numbers between 2 and 10 (i.e., 2^2 + 4^2 + 6^2 + 8^2 + 10^2).
Solving the Problem in C++
C++ is a popular language used for competitive programming and algorithmic problem-solving. Solving the Even Square problem in C++ involves iterating over the given range of numbers, checking if the current number is even, squaring the number if it is even, and adding it to a running total. Here’s the C++ code to solve the Even Square problem:
#include <iostream> using namespace std; int main() { int a,n; cin>>n; for(int i=0;i<n;i++) { cin>>a; if(a>0) { if(a%2==0) cout<<"EVEN POSITIVE"<<endl; else cout<<"ODD POSITIVE"<<endl; } else if(a<0) { if(a%2==0) cout<<"EVEN NEGATIVE"<<endl; else cout<<"ODD NEGATIVE"<<endl; } else cout<<"NULL"<<endl; } return 0; }
Solving the Problem in C
C is another popular language used for competitive programming and algorithmic problem-solving. The Even Square problem can be solved in C using the same logic as in C++. Here’s the C code to solve the Even Square problem:
#include <stdio.h> int main() { int a, b, sum = 0; scanf("%d %d", &a, &b); for(int i = a; i <= b; i++) { if(i % 2 == 0) { sum += i * i; } } printf("%d\n", sum); return 0; }
Solving the Problem in Python
Python is a popular language used for data science, machine learning, and algorithmic problem-solving. The Even Square problem can also be solved in Python using a similar loop-based approach. Here’s the Python code to solve the Even Square problem:
a, b = map(int, input().split()) sum = 0 for i in range(a, b + 1): if i % 2 == 0: sum += i * i print(sum)
Performance Optimization
While the above code solutions are straightforward, there are a few tips and tricks that can be used to optimize the performance of the code. Here are some ways to optimize the code:
Using bitwise operations instead of modulo operator
Instead of using the modulo operator to check if a number is even, we can use bitwise operations. For example, to check if a number is even, we can use the following code: if((i & 1) == 0)
.
previous problem: Beecrowd 1026 To Carry or not to Carry Solution
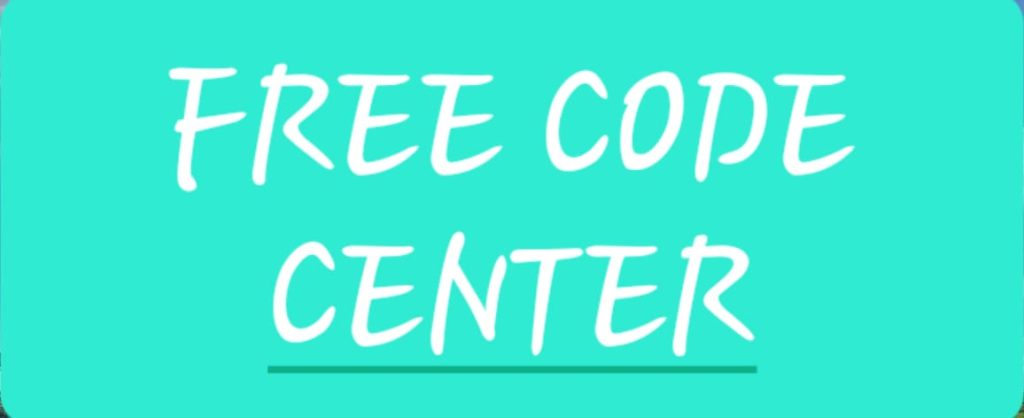