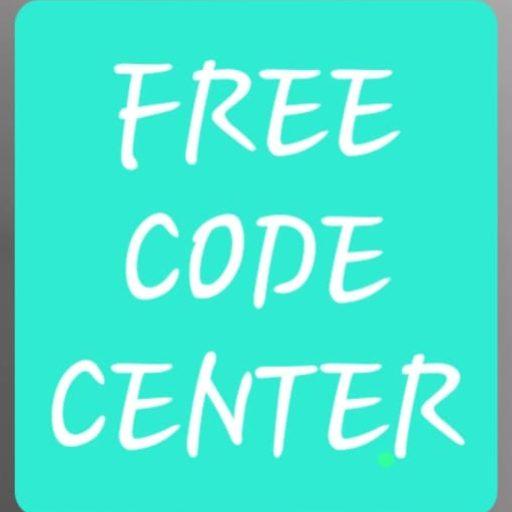
What is an array in C?
An array in C is a collection of elements of the same data type, arranged in contiguous memory locations. Each element of an array can be accessed by an index, which represents its position within the array.
Arrays in C are declared by specifying the data type of the elements in the array, followed by the name of the array, and the number of elements in the array, enclosed in square brackets. For example, the following statement declares an array of 5 integers:
int myArray[5];
The first element of the array is accessed using the index 0, and the last element is accessed using the index n-1, where n is the number of elements in the array. For example, to assign a value of 10 to the first element of the array, we can use the following statement:
myArray[0] = 10;
Arrays can be used in a variety of ways in C, such as storing data, passing arguments to functions, and implementing algorithms.
How to input the array in C?
#include <stdio.h> int main() { int arr[5]; // Declare an array of 5 integers int i; // Read in each element of the array from the user for (i = 0; i < 5; i++) { printf("Enter element %d: ", i); scanf("%d", &arr[i]); } // Print out the elements of the array printf("The elements of the array are: "); for (i = 0; i < 5; i++) { printf("%d ", arr[i]); } return 0; }
In this example, we declare an array of 5 integers and use a loop to read in each element of the array from the user using the scanf()
function. We then use another loop to print out the elements of the array using the printf()
function.
Note that we use the &
operator when reading in each element of the array with scanf()
. This is because the scanf()
function requires the memory address of the variable where the input should be stored.